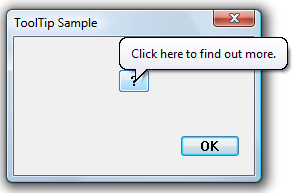
In the Windows XP login screen, the password text box will warn you with a balloon tooltip if you accidentally turn Caps Lock on. The balloon tooltip appears to be a Windows tooltip common control with the TTS_BALLOON
style.
To replicate this functionality, I decided to write a function called ShowMsgBalloon()
which, given a control and the various balloon tooltip parameters, creates and shows the balloon tooltip below the control.
The key insight to making ShowMsgBallon()
work as intended was to use the TTF_TRACK
option to create a tracking tooltip. This will immediately show the tooltip without requiring the user to position the mouse over the control. The main downside to using TTF_TRACK
is that the tooltip will not move with the control if the window is moved; you need to manually move the tooltip using TTM_TRACKPOSITION
as required. One could probably make this automatic by subclassing the tooltip’s parent control and handling WM_WINDOWPOSCHANGED
messages.
Here is the source code to ShowMsgBalloon()
. When you are done with the balloon, call DestroyWindow()
on the returned HWND. Note: you may want your application to use comctl32.dll version 6 as it will lead to a nicer visual style, including a close button.
|
|
Update 2008-11-01 3:08PM: If you are targeting comctl32.dll version 6 or later, I recommend using the EM_SHOWBALLOONTIP
message. Comctl32.dll version 6 or later also automatically shows the caps lock warning balloon for edit boxes with the ES_PASSWORD
window style.