Exploring the .NET CoreFX Part 4: The Requires Convenience Class
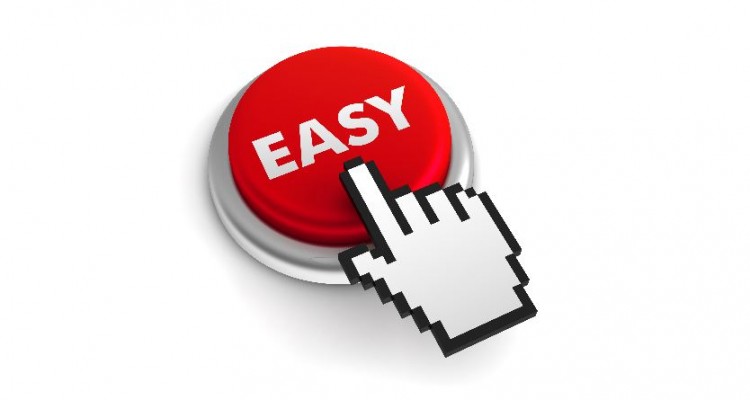
This is part 4/17 of my Exploring the .NET CoreFX series.
The System.Collections.Immutable project in the .NET CoreFX includes a convenience class called Requires, which looks like:
|
|
This allows other methods to write code like:
|
|