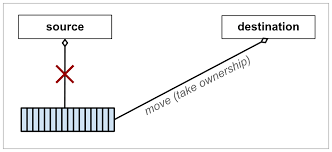
After trying out the Movable<TResource>
type from Move Semantics for IDisposable
I discovered a fatal flaw in its implementation: it is incompatible with struct
memberwise copy semantics.
After trying out the Movable<TResource>
type from Move Semantics for IDisposable
I discovered a fatal flaw in its implementation: it is incompatible with struct
memberwise copy semantics.
See also Move Semantics for IDisposable Part 2
C++ 11 introduced the concept of move semantics to model transfer of ownership.
Rust includes transfer of ownership as a key component of its type system.
C# could benefit from something similar for IDisposable
types. This blog
post explores some options on how to handle this.
This blog post series follows my development of a D3-based latency heatmap visualization.
This blog post series shows how to perform data-driven code generation of unit tests in various different languages and testing frameworks.
When the .NET core framework was first released as open source in 2015, I spent a few weeks reading the source code and looking for tricks and techniques that I thought were interesting or novel. This blog post shares some of the things I found along the way.
This is part 17/17 of my Exploring the .NET CoreFX series.
Microsoft’s .NET Core team has posted a videotaped API review session where they show how they review API enhancement suggestions. I thought the video was quite educational.
This is part 16/17 of my Exploring the .NET CoreFX series.
While .NET has historically been limited to Windows machines, Mono notwithstanding, the introduction of the cross-platform .NET Core runtime has introduced the possibility of running .NET Core applications on Unix machines. With this possibility, developers may have the need of writing platform-specific code.
One way to write platform-specific code is:
interface
, as we will be using compile-time rather than run-time polymorphism.An example from the .NET Core is the System.Console.ConsolePal
class from the System.Console
library. The library includes two implementations of this class:
This is part 15/17 of my Exploring the .NET CoreFX series.
While C# supports type inference for generic methods, it does not support type inference for constructors. In other words, while this code works:
|
|
This code does not:
Read more...This is part 14/17 of my Exploring the .NET CoreFX series.
Back in 2013, Immo Landwerth and Andrew Arnott recorded a Going Deep video called Inside Immutable Collections which describes how and why System.Collections.Immutable is built the way it is. It’s great background material to understand System.Collections.Immutable.
This is part 13/17 of my Exploring the .NET CoreFX series.
Most implementations of IList
, including System.Collections.Generic.List
, are dynamic arrays. System.Collections.Immutable.ImmutableList
is different – it is an AVL tree. This results in significantly different performance characteristics:
List |
ImmutableList |
|
---|---|---|
Indexing | O(1) | O(log n) |
Append | O(1) average, O(n) worst-case | O(log n) |
Insert at arbitrary index | O(n) | O(log n) |
Remove | O(n) | O(log n) |
Memory layout | Contiguous for value types | Non-contiguous |
The data structure behind ImmutableList
was likely chosen so that modifications to the list are non-destructive and require minimal data copying.