Exploring the .NET CoreFX Part 12: Aggressive Inlining
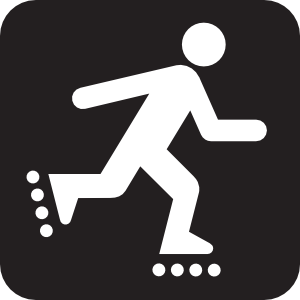
This is part 12/17 of my Exploring the .NET CoreFX series.
In C++, the inline
keyword allows a developer to provide a hint to the compiler that a particular method should be inlined. C# has the identical ability but uses an attribute instead:
|
|
In System.Collections.Immutable, this attribute is used highly selectively – only once, in fact.
Recommendations
- In rare cases, consider using
MethodImpl(MethodImplOptions.AggressiveInlining)
to suggest to the .NET runtime that a particular method should be inlined.